Integrate your WPF
Integrate your WPF into your store and display it to your customers at checkout.
When you integrate and display the WPF to your customers, you create a payment session through which your customers can pay using the emerchantpay Genesis gateway. If you have an existing storefront, integrate the WPF into your existing platform to create a payment session whenever a customer checks out.
Integrating the emerchantpay WPF into an existing storefront requires connecting to the emerchantpay Genesis gateway using the WPF API. You should have a working knowledge of web programming languages, HTTP methods in XML and JSON formats, and UTF8 encoding. Genesis SDK libraries with code samples are available for integrating with the gateway in a range of languages.
For a solution that requires less development overhead, see emerchantpay’s custom shopping cart plugins.Prerequisites
To interact with the Genesis gateway, provide your API credentials using standard HTTP Basic Authentication. Your API credentials are found in the Merchant account creation email you received from emerchantpay support, or by logging in to Genesis.
To get your API credentials in Genesis:
PRODUCTION
.
- Log in to Genesis.
- In the navigation menu, select Configuration > Merchants.
- Next to your merchant name, select Terminals.
- Select the Terminal through which you would like to process.
- The Api access section lists the Api login and Api password fields.
- Send your API credentials alongside your initial Create method request:
emerchantpay recommends you authenticate using HTTP Basic Authentication whenever possible. However, you may need to authenticate through other methods depending on the language library you use.
Example of passing API credentials as a file:<?php
use \Genesis\Config as GenesisConfig;
// Point to the directory containing your configuration file
GenesisConfig::loadSettings(‘/path/to/config.ini’);
Example of passing API credentials individually:
import com.emerchantpay.gateway.api.constants.Endpoints;
import com.emerchantpay.gateway.api.constants.Environments;;
import com.emerchantpay.gateway.util.Configuration;
// Specify environment and endpoint
Configuration configuration = new Configuration(Environments.STAGING, Endpoints.EMERCHANTPAY);
// Add your API authentication credentials
configuration.setUsername(“{YOUR_USERNAME}”);
configuration.setPassword(“{YOUR_PASSWORD}”);
1. Send an initial Create method request
Create an endpoint from your server that connects to the Genesis gateway and requests a WPF that is displayed to your customer. The endpoint passes information to the Gateway like the transaction amount, currency, customer information, and type of payment.
Example of passing transaction information: <?xml version=”1.0″ encoding=”UTF-8″?>
<wpf_payment>
<transaction_id>119643250547501c79d8295</transaction_id>
<usage>40208 concert tickets</usage>
<description>You are about to buy 3 tickets.</description>
<notification_url>https://www.example.com/notification</notification_url>
<return_success_url>http://www.example.com/success</return_success_url>
<return_failure_url>http://www.example.com/failure</return_failure_url>
<return_cancel_url>http://www.example.com/cancel.html</return_cancel_url>
<return_pending_url>http://www.example.com/payment-pending.html</return_pending_url>
<amount>100</amount>
<currency>USD</currency>
<consumer_id>123456</consumer_id>
<customer_email>jsmith@example.com</customer_email>
<customer_phone>+001234567890</customer_phone>
<remember_card>true</remember_card>
<lifetime>60</lifetime>
Example of passing billing information:
<billing_address>
<first_name>John</first_name>
<last_name>Smith</last_name>
<address1>123 Main St.</address1>
<zip_code>12345</zip_code>
<city>London</city>
<country>UK</country>
</billing_address>
Example of passing transaction type details:
<transaction_types>
<transaction_type moto=”true” name=”authorize” fx_rate_id=”123″/>
<transaction_type crypto=”true” name=”sale” fx_rate_id=”123″/>
</transaction_types>
2. Receive a response
You will receive a Success or Error response to your Create method request. A Success response will contain a redirect_url
that contains a unique URL address of the payment session for you to redirect your customer to.
<?xml version=”1.0″ encoding=”UTF-8″?>
<wpf_payment>
<transaction_type>wpf_create</transaction_type>
<status>new</status>
<mode>live</mode>
<transaction_id>119643250547501c79d8295</transaction_id>
<consumer_id>123456</consumer_id>
<unique_id>44177a21403427eb96664a6d7e5d5d48</unique_id>
<technical_message>Transaction successful!</technical_message>
<message>Transaction successful!</message>
<redirect_url>https://staging.wpf.emerchantpay.net/en/v2/payment/c7e32c1e9d1</redirect_url>
<timestamp>2022-05-24T17:25:08Z</timestamp>
<amount>100</amount>
<currency>USD</currency>
</wpf_payment>
3. Redirect your customer
Redirect your customer to the unique URL address of the payment session that you received in the redirect_url
parameter of your WPF Create method request. Your customer sees your WPF hosted on the emerchantpay servers.
Depending on how your Merchant account is configured, your customer will see a range of payment methods through which to pay for the transaction.
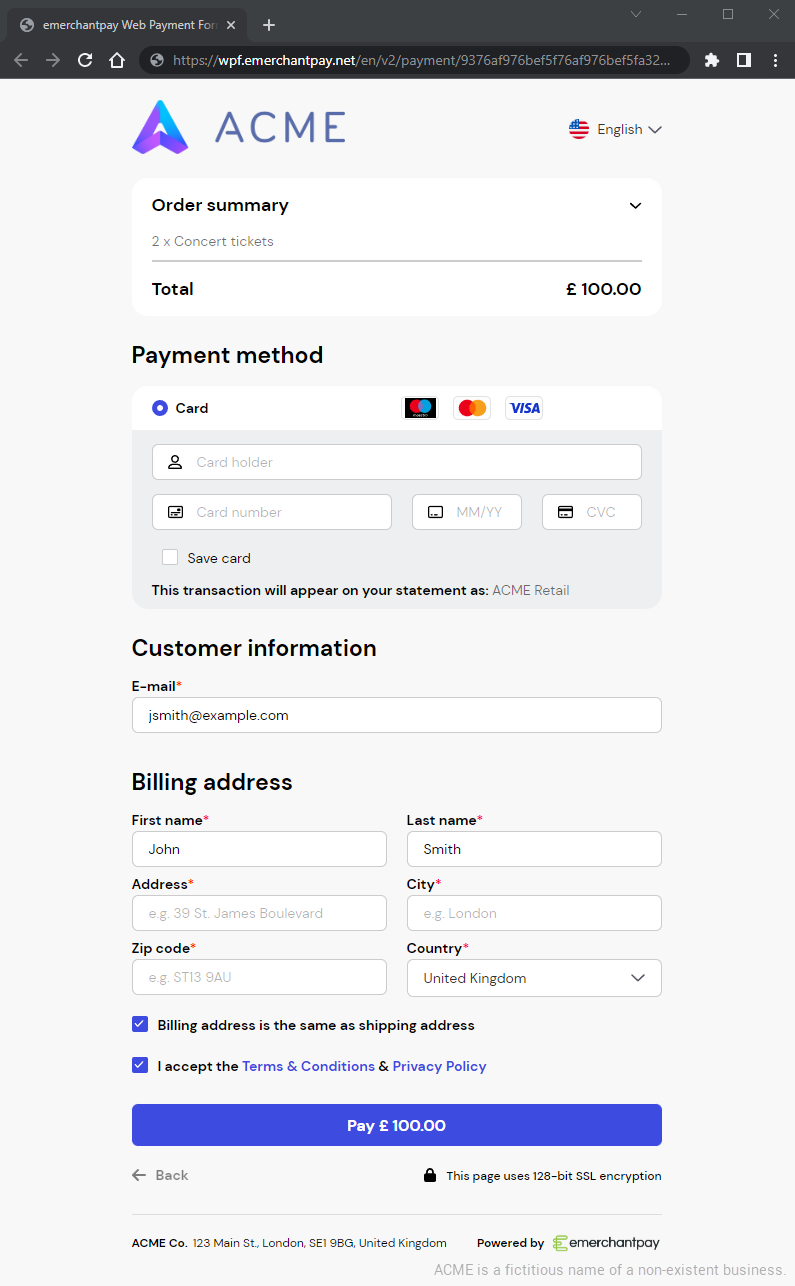
4. Show a success page
After your customer submits their payment, they will be redirected back to your storefront. Here they will see a success page, failure page, or another type of status page that you host on your server. Status pages are submitted as part of your initial Create method request.
<notification_url>https://www.example.com/notification</notification_url>
<return_success_url>http://www.example.com/success</return_success_url>
<return_failure_url>http://www.example.com/failure</return_failure_url>
<return_cancel_url>http://www.example.com/cancel.html</return_cancel_url>
<return_pending_url>http://www.example.com/payment-pending.html</return_pending_url>
notification_url
is the address where you receive HTTP POST notifications from the gateway about each transaction.
5. Verify the payment
In addition to the Success response to your Create request, you will receive a HTTP POST notification for each payment session from the Genesis gateway to the notification_url
that you supplied with the Create method request.
The wpf_status
parameter shows the result of the transaction.
signature=c5219b3d385e74496b2b48a5497b347e102849f10eacd25b062f823b
&payment_transaction_transaction_type=sale3d
&payment_transaction_terminal_token=e9fd7a957845450fb7ab9dccb498b6e1f6e1e3aa
&payment_transaction_unique_id=bad08183a9ec545daf0f24c48361aa10
&payment_transaction_amount=500
&wpf_transaction_id=mtid201104081447161135536962
&wpf_status=approved
&wpf_unique_id=26aa150ee68b1b2d6758a0e6c44fce4c
&consumer_id=123456
&payment_transaction_token=ee946db8-d7db-4bb7-b608-b65b153e127d
¬ification_type=wpf
&eci=05
&payment_transaction_avs_response_code=5I
&payment_transaction_avs_response_text=Response+provided+by+issuer+processor%3B+Address+information+not+verified
&payment_transaction_cvv_result_code=M
&authorization_code=005645
&retrieval_reference_number=016813015184
&scheme_response_code=00
&scheme_transaction_identifier=MCS267BG0
&scheme_settlement_date=1103
&threeds_authentication_flow=payer_authentication
&threeds_target_protocol_version=1
&threeds_concrete_protocol_version=1
Use the signature
field to verify that the notification has been sent by the Genesis gateway. Each session signature is generated by combining the wpf_unique_id
and your API password, and generating a SHA-512 hash function of the combined string.
signature
= SHA-512 hash value of <wpf_unique_id><API password>
wpf_unique_id | API password | signature |
---|---|---|
26aa150ee68b1b2d6758a0e6c44fce4c | 50fd87e65eb415f42fb5af4c9cf497662e00b785 | c5219b3d385e74496b2b48a549 |
3f760162ef57a829011e5e2379b3fa17 | 50fd87e65eb415f42fb5af4c9cf497662e00b785 | 14519d0db2f7f8f407efccc9b099 |
After you have verified the notification state and validity, render an XML page containing the transaction’s unique id to acknowledge that you have received the notification.
Example of a notification reply you use to confirm a notification:<?xml version=”1.0″ encoding=”UTF-8″?>
<notification_echo>
<wpf_unique_id>3f760162ef57a829011e5e2379b3fa17</wpf_unique_id>
</notification_echo>
6. Optional: Reconcile the transaction
Send a reconcile request to the Genesis gateway to get information about a transaction. Reconcile a transaction to verify a successful payment, or to get information about a transaction that has timed out, returned an error, or is in chargeback.
Reconcile a transaction by sending request that contains the unique_id
returned by the Create method request.
<?xml version=”1.0″ encoding=”UTF-8″?>
<wpf_reconcile>
<unique_id>26aa150ee68b1b2d6758a0e6c44fce4c</unique_id>
</wpf_reconcile>